WEB SDK
Web-browser (Chrome, Firefox, etc.) push input format
Api key for app is a GCM/FCM server key (required for Chrome push and for other browsers which use GCM, e.g. Opera).
Example transform template:
[
{
"operation": "shift",
"spec": {
"url": "data.url",
"*": "&"
}
},
{
"operation": "default",
"spec": {
"icon": "icon.png",
"data": { "url": "http://push.delivery" },
"requireInteraction": "true"
}
}
]
This template transforms "url":"link" to "data":{url:"link"} (as required for notification options),
writes all other key-value pairs as is and adds default elements to resulting json.
The API for showing a notification in browser (javascript):
<ServiceWorkerRegistration>.showNotification(<title>, <options>);
Title and options could be declared and used as following:
const notificationTitle = 'Title';
const notificationOptions = {
"title":"eThinking Push Demo",
"body": "Body text line 1 \n Body text line 2",
"data": { "url": "http://push.delivery" },
...
};
...
self.registration.showNotification(notificationTitle, notificationOptions);
Thus if the above described template and demo service worker (see source code) are used,
then the outgoing json should contain following options:
The list of common notification options:
* title - notification's title * body - notification's body text * icon - url to icon image * image - url to body image * url - url will opened in a new window by click on the notification * requireInteraction - if true, then the notification will not disappear until user action * badge - small monochrome icon * actions - array of actions / buttons (see example 2)
Other options:
* timestamp * sound * vibrate * dir * tag * renotify * silent
More options and info on https://developer.mozilla.org/en-US/Add-ons/WebExtensions/API/notifications/NotificationOptions
and https://developers.google.com/web/fundamentals/push-notifications/display-a-notification
Example push message 1 ( title, icon, body and image ):
{
"title":"eThinking Push Demo",
"icon":"icon.png",
"url":"http://push.delivery",
"body":"Good morning!",
"requireInteraction":"true",
"image":"https://imageUrl"
}
notification view in Chrome:
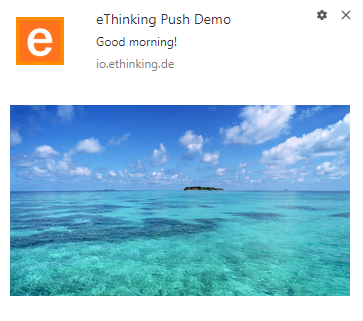
"Image" and "Require interaction" options work only in Chrome at the moment (tested on Google Chrome 60, Firefox 55, Opera 47). An "Image" option requires "https" url.
Example push message 2 ( title, icon, body and buttons ):
{
"title":"Demo title",
"icon":"iconFile.png",
"body":"Hello!",
"requireInteraction":"true",
"actions": [
{"action": "like", "title": "Like", "icon": "https://example/like.png"},
{"action": "reply", "title": "Reply", "icon": "https://example/reply.png"}
]
}
notification view in Chrome:
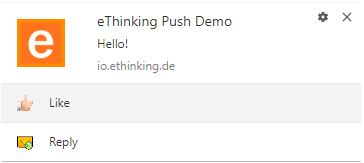
Actions should be handled in service worker:
self.addEventListener('notificationclick', function (event) { event.notification.close(); let clickResponsePromise = Promise.resolve(); if (event.action === 'like') { clients.openWindow("http://..."); } else if (event.action === 'reply') { clients.openWindow("http://..."); } event.waitUntil(Promise.all([clickResponsePromise])); });
Javascript API
Overview
This API documentation covers usage of the Javascript library to receive push notifications
To use this library you need to have a project setup by ethinking. Please get in contact if you don’t have access to the Admin UI of the push library.
Setup of library
To initiate the library you need to do the following
<script src="<libraryLink>"></script> (1)
1 | Library link provided with customer onboarding. |
let push = new ethinkingPush(
messageSenderId, (1)
projectName, (2)
serviceWorkerPath (3)
);
1 | Firebase messengerId, retrieved from the firebase console |
2 | Project Name, provided by us beforehand |
3 | Path to your service worker, can be anything but needs .js ending: firebase_service_worker.js |
Functions
init()
Initializes the push for a certain app. For the browser push Firefox and Chrome use the same app. All infos are coming from the Admin UI
push.init({
appId: '123', (1)
user: 'api-test-123', (2)
password: '12345657890' (3)
});
To get any of the information, go to your Admin UI, open an App
1 | String - AppId |
2 | String - AppUser |
3 | String - AppPassword |
getTags()
Gets tags from the push backend, depending on the status of the user it returns subscribed and all Tags
getTags() returns a promise, the objects represents all tags from your app and subscribed apps depending on the subscription status of the user |
push.getTags().then(result => {
// TODO show tags here in a list
});
The format of the promise will be an Array with the following structure:
[
{
id: 111,
name: 'Test Tag',
subscribed: false
},
{
id: 111,
name: 'Test Tag',
subscribed: false
}
]
registerTags(tags)
Register several or a single tag to the current DeviceId
The parameter tags can be a number or an Array
|
push.registerTags(123);
push.registerTags([123, 456]);
unregisterTags(tags)
Unregister several or a single tag to the current DeviceId
The parameter tags can be a number or an Array
|
push.unregisterTags(123);
push.unregisterTags([123, 456]);
subscribe(tags)
Subscribes the user and gets a deviceId necessary to update Tags. Requests the permission if none is given, gets deviceToken and stores it in localStorage
The parameter tags can be a number or an Array
|
push.subscribe([123, 456]);